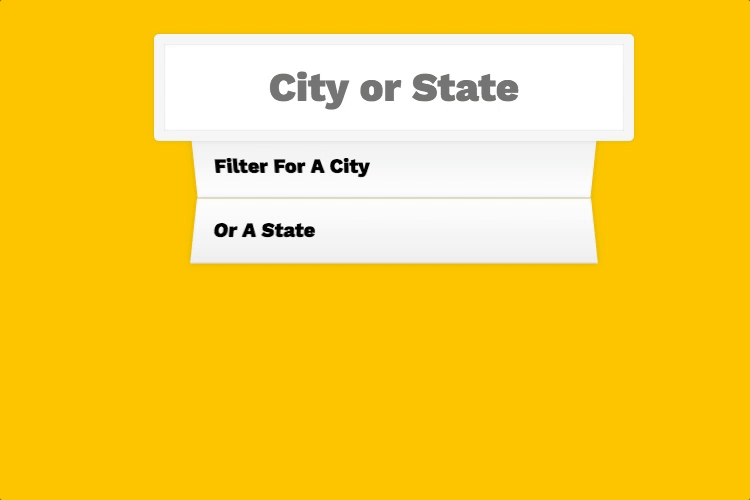
JS 30 Day 6
Live Demo
The Task:
Make a input field with a "type ahead" feature. When typing into the field it will match and filter into a subset of the entire JSON.
Thoughts:
Using fetch() is awesome! Which is supported by all browsers according to MDN except for Internet Explorer. But there is apparently a polyfill to make it work on the unsupported browsers. Using Fetch Below is the use of fetch() used in this project.
const endpoint = 'https://gist.githubusercontent.com/Miserlou/c5cd8364bf9b2420bb29/raw/2bf258763cdddd704f8ffd3ea9a3e81d25e2c6f6/cities.json';
// fetch the URL which will return a blob
// The blob could be any kind of data type
// Since we know its json we use the built in method
// We then need another promise and we have the raw data
// we call push onto our array we made and use the ES6 spread to
// insert each city into its own index in our array
fetch(endpoint).then(blob => blob.json().then(data => cities.push(...data)))
One thing I did notice, if a capital is typed, it will change where ever that letter occurs to be capital. For example, if a capital "R" is typed. it will show up as New YoRk in the results. I believe this would require the regex and replace methods to be refactored a bit. Below is the whole javascript file.
'use strict';
const endpoint = 'https://gist.githubusercontent.com/Miserlou/c5cd8364bf9b2420bb29/raw/2bf258763cdddd704f8ffd3ea9a3e81d25e2c6f6/cities.json';
const cities = [];
// fetch the URL which will return a blob
// The blob could be any kind of data type
// Since we know its json we use the built in method
// We then need another promise and we have the raw data
// we call push onto our array we made and use the ES6 spread to
// insert each city into its own index in our array
fetch(endpoint).then(blob => blob.json().then(data => cities.push(...data)))
function findMatch(wordToMatch, cities){
return cities.filter(place=> {
const regex = new RegExp(wordToMatch,'gi');
return place.city.match(regex) || place.state.match(regex);
});
}
function numberWithCommas(x){
return x.toString().replace(/\B(?=(\d{3})+(?!\d))/g,',');
}
function displayMatches(){
const matchArray = findMatch(this.value, cities);
const html = matchArray.map(place => {
const regex = new RegExp(this.value, 'gi');
const cityName = place.city.replace(regex, `${this.value}`);
const stateName = place.state.replace(regex, `${this.value}`);
return `
${cityName}, ${stateName}
${numberWithCommas(place.population)}
`;
}).join('');
suggestions.innerHTML = html;
}
const searchInput = document.querySelector('.search');
const suggestions = document.querySelector('.suggestions');
searchInput.addEventListener('change', displayMatches);
searchInput.addEventListener('keyup', displayMatches);
Here is the source for day 6.